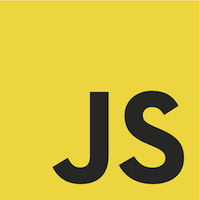
Fetch Data from an API
Learn how to fetch data from an API using the Fetch API in JavaScript. This snippet demonstrates making a GET request and handling the response asynchronously, perfect for modern web development.
A collection of useful Javascript code snippets.
Learn how to fetch data from an API using the Fetch API in JavaScript. This snippet demonstrates making a GET request and handling the response asynchronously, perfect for modern web development.
Learn how to implement debouncing in JavaScript to optimize input handling. This snippet shows how to debounce a function, useful for improving search input performance and reducing server load.
Learn how to generate unique identifiers using JavaScript. This snippet demonstrates creating a version 4 UUID with the Web Cryptography API, ensuring high uniqueness and security.
Learn how to deep clone an object in JavaScript using structured cloning. This snippet ensures all nested objects and arrays are cloned, preventing changes to the original object.
Learn how to throttle scroll events in JavaScript to optimize performance. This snippet demonstrates limiting the rate at which a scroll event handler is executed, ensuring smoother scrolling experiences.
Learn how to sort an array of objects by a specific property in JavaScript. This snippet demonstrates using the sort method with a custom comparison function to order objects by their properties.
Learn how to debounce click events in JavaScript to prevent multiple submissions and redundant function calls. This snippet demonstrates using a custom debounce function for handling click events.
Learn how to find the largest number in an array using JavaScript. This snippet demonstrates using the reduce method to iterate through an array and determine the largest number.
Learn how to merge two arrays without duplicates using JavaScript. This snippet demonstrates using the Set object and the spread operator to combine arrays and remove duplicate values.
Learn how to calculate the sum of array elements using JavaScript. This snippet demonstrates using the reduce method to iterate through an array and calculate the total sum of its elements.
Learn how to validate email format using JavaScript. This snippet demonstrates using a regular expression to check if an email address is correctly formatted.
Learn how to shuffle an array using JavaScript. This snippet demonstrates the Fisher-Yates Shuffle algorithm for randomizing the order of array elements.
Learn how to get query parameters from a URL using JavaScript. This snippet demonstrates using the URL and URLSearchParams objects to parse and retrieve query parameters.
Learn how to flatten a nested array using JavaScript. This snippet demonstrates using the flat method to recursively flatten nested arrays to a specified depth.
Learn how to remove duplicates from an array using JavaScript. This snippet demonstrates using the Set object and the spread operator to create a unique array.
Learn how to format a date to a readable string using JavaScript. This snippet demonstrates using the toLocaleDateString method to format dates according to specified locale and options.
Learn how to convert an array to a CSV string using JavaScript. This snippet demonstrates converting an array of objects to a CSV format, useful for exporting data and generating reports.
Learn how to copy text to the clipboard using JavaScript. This snippet demonstrates using the Clipboard API to write text to the clipboard, providing a simple and secure way to copy information.
Learn how to check if an element is in the viewport using JavaScript. This snippet demonstrates using the IntersectionObserver API to detect when an element enters or exits the viewport.
Learn how to capitalize the first letter of each word in a string using JavaScript. This snippet demonstrates using string methods to format text for improved readability and presentation.
Learn how to find unique elements in an array using JavaScript. This snippet demonstrates using the Set object and the spread operator to create a unique array.
Learn how to convert RGB values to a hex color code using JavaScript. This snippet demonstrates using the toString and padStart methods to create hex color codes from RGB values.
Learn how to remove a property from an object using JavaScript. This snippet demonstrates using the delete operator to dynamically modify object structures.
Learn how to convert an array to an object using JavaScript. This snippet demonstrates using the reduce method to transform key-value pairs into an object for better data organization.
Learn how to deep merge two objects using JavaScript. This snippet demonstrates using a recursive approach to combine nested properties and handle complex data structures.
Learn how to throttle function execution using JavaScript. This snippet demonstrates limiting the rate at which a function is called, improving performance and reducing load on the browser.
Learn how to create a deep copy of an array using JavaScript. This snippet demonstrates using JSON methods to duplicate nested arrays and objects, preventing changes to the copied array from affecting the original.
Learn how to get a random item from an array using JavaScript. This snippet demonstrates using the Math.random and Math.floor methods to select a random element from an array.
Learn how to count the occurrences of elements in an array using JavaScript. This snippet demonstrates using the reduce method to create a frequency distribution object that maps each element to its count.
Learn how to group array elements by a property using JavaScript. This snippet demonstrates using the reduce method to create an object where each key corresponds to a property value and its associated array of elements.
Learn how to flatten an array of arrays using JavaScript. This snippet demonstrates using the flat method to concatenate nested arrays into a single array.
Learn how to sort an array of objects by multiple properties using JavaScript. This snippet demonstrates using the sort method and comparison functions to organize data by multiple criteria.
Learn how to generate a random password using JavaScript. This snippet demonstrates using the Math.random method and a comprehensive character set to create secure passwords.
Learn how to convert a string to camel case using JavaScript. This snippet demonstrates using string methods and array operations to format text in camel case, useful for variable names and text formatting.
Learn how to filter an array by a search term using JavaScript. This snippet demonstrates using the filter and includes methods to narrow down data based on user criteria, useful for search functionality.
Learn how to calculate the factorial of a number using JavaScript. This snippet demonstrates both iterative and recursive approaches to calculate factorials, useful for algorithms and mathematical computations.
Learn how to detect if a user is on a mobile device using JavaScript. This snippet demonstrates using the user agent string and regular expressions to identify mobile devices and apply conditional logic.
Learn how to create a simple event emitter using JavaScript. This snippet demonstrates building an event emitter class to handle custom events, register listeners, and manage event-driven interactions.
Learn how to generate a random string using JavaScript. This snippet demonstrates using the Math.random method and a comprehensive character set to create unique and random strings.
Learn how to debounce scroll events in JavaScript to optimize performance. This snippet demonstrates using a custom debounce function to limit the rate at which a scroll event handler is executed.
Learn how to convert CSV data to an array of objects using JavaScript. This snippet demonstrates using string methods and array operations to transform CSV data into a structured format for processing.
Learn how to calculate the Fibonacci sequence using JavaScript. This snippet demonstrates both iterative and recursive approaches to generate Fibonacci numbers, useful for algorithms and problem-solving.
Learn how to deep clone an object with circular references using JavaScript. This snippet demonstrates using WeakMap and recursion to handle circular references and ensure correct cloning of nested data.
Learn how to create a simple image carousel using JavaScript. This snippet demonstrates using DOM manipulation and event handling to build an interactive and responsive image carousel.
Learn how to calculate the sum of array elements using JavaScript. This snippet demonstrates using the reduce method to sum values in an array, useful for data analysis and mathematical computations.
Learn how to check if a number is a prime using JavaScript. This snippet demonstrates an efficient approach to determine the primality of a number, useful for algorithms and problem-solving.
Learn how to create a countdown timer using JavaScript. This snippet demonstrates using the setInterval method and DOM manipulation to create a dynamic and interactive countdown timer.
Learn how to calculate the average of array elements using JavaScript. This snippet demonstrates using the reduce method to sum values in an array and calculate their average, useful for data analysis and mathematical computations.
Learn how to remove elements from an array based on a condition using JavaScript. This snippet demonstrates using the filter method to select elements that do not satisfy a condition, effectively removing them from the array.
Learn how to convert a date to an ISO string using JavaScript. This snippet demonstrates using the toISOString method to represent date and time information in a standardized format.
Learn how to merge two arrays and remove duplicates using JavaScript. This snippet demonstrates using the Set object and the spread operator to combine arrays and ensure uniqueness.
Learn how to convert JSON data to CSV format using JavaScript. This snippet demonstrates using the map and join methods to transform JSON data into a CSV string for exporting and reporting.
Learn how to count the number of vowels in a string using JavaScript. This snippet demonstrates using regular expressions and string methods to match and count vowels in a string.
Learn how to validate phone number format using JavaScript. This snippet demonstrates using a regular expression to check if a phone number matches a specified pattern, ensuring accurate and correctly formatted input.
Learn how to calculate the Greatest Common Divisor (GCD) of two numbers using JavaScript. This snippet demonstrates using the Euclidean algorithm to determine the GCD, useful for mathematical and algorithmic problems.
Learn how to check if a string contains a substring using JavaScript. This snippet demonstrates using the includes method to determine the presence of a substring within a string, useful for searching and filtering tasks.
Learn how to generate a UUID using JavaScript. This snippet demonstrates using random numbers and hexadecimal formatting to create a unique and compliant UUID for various use cases.
Learn how to convert Fahrenheit to Celsius using JavaScript. This snippet demonstrates using the conversion formula to accurately convert temperatures from Fahrenheit to Celsius, useful for various temperature-related tasks.
Learn how to generate a random hex color using JavaScript. This snippet demonstrates generating a random hex color code for use in dynamic themes, backgrounds, and more.
Learn how to check if a string is a palindrome using JavaScript. This snippet demonstrates using regular expressions and string methods to determine if a string reads the same backward as forward.
Learn how to detect the browser and operating system using JavaScript. This snippet demonstrates using the user agent string and regular expressions to identify the user's environment.
Learn how to fetch and display JSON data using JavaScript. This snippet demonstrates using the Fetch API to retrieve data from an API and display it dynamically on a web page.
Learn how to implement drag and drop functionality using JavaScript. This snippet demonstrates using the HTML5 Drag and Drop API to create interactive and user-friendly interfaces.
Learn how to sort table rows using JavaScript. This snippet demonstrates sorting table rows based on the content of a specific column to create sortable and interactive data tables.
Learn how to implement infinite scroll using JavaScript. This snippet demonstrates using the IntersectionObserver API and Fetch API to load additional content as the user scrolls down the page.
Learn how to convert a string to title case using JavaScript. This snippet demonstrates using string methods and array operations to capitalize the first letter of each word in a string.
Learn how to implement a countdown timer using JavaScript. This snippet demonstrates using the setInterval method and DOM manipulation to create a dynamic and interactive countdown timer.
Learn how to check if an object is empty using JavaScript. This snippet demonstrates using the Object.keys method to determine whether an object has any own enumerable properties.