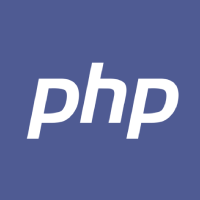
User Authentication via Session
Learn how to implement a secure user authentication system using PHP sessions. This guide covers session management, security practices, and error handling.
A collection of PHP code snippets for web development.
Learn how to implement a secure user authentication system using PHP sessions. This guide covers session management, security practices, and error handling.
Securely connect to a database using PHP Data Objects (PDO) with comprehensive error handling. Learn to implement a reusable database connection strategy in PHP.
Learn how to send emails using PHPMailer with SMTP. This guide covers configuration, HTML emails, and robust error handling for reliable email functionality in PHP.
Implement secure file upload functionality in PHP with checks for file type, size, and error handling. Learn best practices for processing user-uploaded files safely.
Efficiently resize images using the GD library in PHP. This guide covers loading, resizing, and saving images while maintaining aspect ratio and handling errors.
Learn how to decode, process, and encode JSON data in PHP. This snippet provides robust error handling and practical examples for API development and data interchange.
Build a simple REST API in PHP. This snippet includes handling of HTTP methods, response codes, and best practices for a lightweight and effective API.
Learn how to use Memcached for caching in PHP to enhance application performance and scalability. This snippet covers setup, basic operations, and error handling.
Implement crucial security measures in PHP web applications. This guide covers input validation, sanitization, security headers, and best practices to mitigate common vulnerabilities.
Automate periodic tasks in PHP applications using cron jobs. Learn to schedule tasks, handle errors, and log activities to ensure smooth and reliable task execution.
Create a backend for a real-time chat system using PHP and WebSockets with the Ratchet library. This guide includes connection handling, message broadcasting, and error management.
Learn how to generate dynamic PDF documents with PHP using the TCPDF library. This guide covers document setup, content addition, and error handling.
Develop a simple PHP-based blog system with CRUD operations. Learn to handle form submissions, use MySQLi with prepared statements, and implement robust error handling.
Ensure the security and integrity of form data in PHP applications with comprehensive input validation and sanitization. Learn best practices for handling user inputs securely.
Implement secure and efficient file downloads in PHP applications. This guide covers setting download headers, handling file access, and ensuring robust error management.
Learn to create and manage secure sessions in PHP applications. This guide covers session setup, security best practices, and comprehensive error handling.
Secure your AJAX requests in PHP applications. This guide covers verifying request authenticity, handling errors, and integrating JSON responses for robust web interactions.
Implement data encryption and decryption in PHP using OpenSSL. Learn to handle keys securely, encrypt/decrypt data, and manage errors effectively for maximum data protection.
Optimize your PHP database queries for improved performance. Learn about using indexes, query caching, prepared statements, and handling errors efficiently.
Secure your RESTful APIs with token-based authentication in PHP. Learn to generate, validate, and manage JWTs for secure and efficient user authentication.
Implement CORS handling in your PHP-based RESTful APIs to manage cross-origin requests securely. Learn how to set up CORS headers and handle preflight requests effectively.
Learn to implement MVC architecture in PHP to enhance application design and maintainability. This guide covers the basics of setting up controllers, models, and views.
Automate email notifications in PHP to enhance user engagement. This guide covers integrating with SMTP servers, handling email delivery errors, and sending rich content emails.
Create a dynamic image gallery in PHP. Learn to automatically fetch and display images from a directory, handle errors, and enhance web page aesthetics with minimal effort.
Set up a secure user login system in PHP with password hashing and session management. This guide includes secure database queries, error handling, and user feedback for optimal security.
Export data from a database to a CSV file in PHP. Learn to connect to a database, execute a query, handle errors, and write data to a CSV for easy analysis and reporting.
Implement SEO-friendly URLs in PHP with .htaccess. Learn to configure URL rewriting for better search engine visibility and user experience.
Integrate Google reCAPTCHA into PHP forms for enhanced security. This guide covers setup, verification, and handling errors to prevent spam and automated submissions.
Generate barcodes in PHP using the TCPDF library. Learn to create barcodes in various formats, embed them in PDF documents, and handle errors effectively.
Import data from CSV files into a MySQL database using PHP. Learn to read, parse, and insert CSV data into a database table with robust error handling.
Implement two-factor authentication in PHP using TOTP. Enhance security by integrating Google Authenticator and handling errors effectively for robust user authentication.
Combine PHP and HTML5 Geolocation API to create location-based services. Learn to capture, send, and process geographical data with robust error handling.
Develop REST API endpoints in PHP to facilitate data exchange and services. This guide covers handling different HTTP methods, returning JSON responses, and managing errors.
Implement error logging and monitoring in PHP to track and resolve issues efficiently. Learn to set up custom error handlers and log errors for improved debugging and maintenance.
Send push notifications from PHP applications to enhance user engagement. This guide covers setting up push notification payloads, connecting to push services, and handling errors.
Implement basic CRUD operations using PDO in PHP. Learn to securely create, read, update, and delete data in a database with robust error handling.
Implement data caching in PHP using Redis to enhance performance and scalability. Learn to connect to Redis, manage cache values, and handle errors effectively.